Thats all well and good but lets do something with it. Since I've already written a post on saving images from flash lets write a little program to take a snapshot of the webcam and save the image to the users desktop!
So lets have a look how this would work:
We already have the following code to create a webcam on the stage -
var CAM:Camera = Camera.getCamera();
import com.JPGEncoder;
This imports the class we'll need to use to create an image. Next, and just below that add this:
We already have the following code to create a webcam on the stage -
var CAM:Camera = Camera.getCamera();
CAM.setQuality(0, 100);
CAM.setMode(550, 400, stage.frameRate );
var VIDEO:Video = new Video(550, 400);
VIDEO.attachCamera(CAM);
addChild(VIDEO);
So lets add an event listener to the stage for when a user clicks on the image, this will later invoke the function to save the image.
stage.addEventListener(MouseEvent.MOUSE_DOWN, save);
function save(e:MouseEvent):void
{
}
The tool I use to encode image data is called AS3 core lib. This tool has a load of classes but the one we want to use is called JPGEncoder.as, and you can download it here.
So lets import that into our movie. First create a folder, call it "com" and drag your newly acquired file into it. This folder should be in the same directory as you .fla and .swf files. Above all of the other code on the first frame write the following:import com.JPGEncoder;
This imports the class we'll need to use to create an image. Next, and just below that add this:
var jpg_encoder:JPGEncoder = new JPGEncoder(100);
This initiates the class and sets the jpg output quality to 100. Of course if you want to output low quality jpegs you could always change this to 50 or something else.
So we have the jpeg encoder, what this does is converts bitmap data into a byte array which your computer will be able to read as a file. So next we'll create a bitmap, this should have the same resolution as your webcam stream (in this case 550x400).
var bitmap:BitmapData(550,400);
Now, within the save function lets draw the VIDEO object into the bitmap data using the draw() function
bitmap.draw(VIDEO);
If you read my previous post on saving from flash you'll already know exactly how to save files, just in case you missed it here it is -
1) Create a file reference object:
fileToSave:FileReference = new FileReference();
2) In your save function encode the jpeg data, we'll use the ByteArray class...
var bytes:ByteArray = jpg_encoder.encode(bitmap);
3) and finally just after that within the save function
fileToSave.save(bytes,"screenshot"+".jpg");
The parameters are data and name, the data being the encoded jpeg and in this case the name is "screenshot.jpg". Note you'll need the extension for your computer to recognise the data as an image.
So here is an example, take a little snap of yourself from your webcam. (The whole file is only 8kb!)
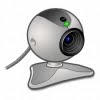
Enjoy guys!
Thanks for the post
ReplyDeleteas3 save bitmap